For this hack, I used the following:
1. A DFRduino Duemilanove (Arduino Compatible) 328 board
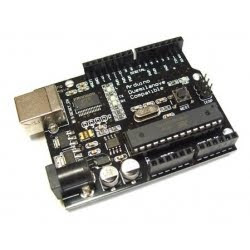
2. DFRobot LCD Shield for Aduino
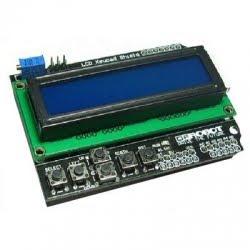
To program the codes, I use the latest Arduino Software, ver 0021, which you can download here: http://arduino.cc/en/Main/Software. This software allows you to write the script as well as compile the codes necessary for the Arduino to run.
Here are the codes I used:
#include <DateTime.h>
#include <DateTimeStrings.h>
#define dt_SHORT_DAY_STRINGS
#define dt_SHORT_MONTH_STRINGS
// simple sketch to display a digital clock on an LCD
// see the LiquidCrystal documentation for more info on this
LiquidCrystal lcd(8, 9, 4, 5, 6, 7);
int backLight = 13; // pin 13 will control the backlight
void setup(){
pinMode(backLight, OUTPUT);
digitalWrite(backLight, HIGH); // turn backlight on. Replace 'HIGH' with 'LOW' to turn it off.
DateTime.sync(DateTime.makeTime(0, 44, 13, 16, 10, 2010)); // sec, min, hour, date, month, year // Replace this with the most current time
}
void loop(){
if(DateTime.available()) {
unsigned long prevtime = DateTime.now();
while( prevtime == DateTime.now() ) // wait for the second to rollover
;
DateTime.available(); //refresh the Date and time properties
digitalClockDisplay( ); // update digital clock
}
}
void printDigits(byte digits){
// utility function for digital clock display: prints preceding colon and leading 0
lcd.print(":");
if(digits < 10)
lcd.print('0');
lcd.print(digits,DEC);
}
void digitalClockDisplay(){
lcd.clear();
lcd.begin(16,2);
lcd.setCursor(3,0);
//lcd.print(DateTimeStrings.dayStr(DateTime.DayofWeek));
if(DateTime.Day <10)
lcd.print('0');
lcd.print(DateTime.Day,DEC);
lcd.print("/");
//lcd.print(DateTimeStrings.monthStr(DateTime.Month));
if(DateTime.Month <10)
lcd.print('0');
lcd.print(DateTime.Month,DEC);
lcd.print("/");
lcd.print((DateTime.Year,DEC)+2000);
//lcd.print(" ");
if(DateTime.Hour <10)
lcd.setCursor(5,1);
lcd.setCursor(4,1);
// digital clock display of current time
lcd.print(DateTime.Hour,DEC);
printDigits(DateTime.Minute);
printDigits(DateTime.Second);
}
Once you have all that done, stack the LCD shield on the Arduino board (make sure the pins line up), plug in the USB cables to the board as well as to your PC, and hit Compile on the Arduino software. The codes will be uploaded and you shall be able to see the result on your LCD screen!
If all goes well, you should be able to see the following:
Note that the keypad on the LCD shield is not functional as I did not include any codes for them to respond to. That itself may be the next hack for me to try!
Bugs/Problems/Feedback/Comments? List them down in the comments and I'll try my best to help you out!
I've the exact component like you which is the DFRobot LCD Shield for Aduino and DFRduino Duemilanove (Arduino Compatible) 328 board and I've installed all the library that are needed by this program, but the result is not the same as you just shown to us. Is there any missing step that I've been missed? Thanks in advance.
ReplyDeleteWhat do you see then?
ReplyDeleteI cant get the DateTime lib to work when it tries to compile it say it can't be found the DateTimeString works both of them are in the libraies directory
ReplyDeleteThis comment has been removed by the author.
DeleteIn your DateTime library, look for the file DateTime.h
ReplyDeleteOpen the file and add the following line
#include < Arduino.h >
Good Luck.
Charles
I am also having trouble getting the DateTime library to work. I am getting the following errors:
ReplyDeleteC:\Users\Will\Downloads\Arduino\arduino-1.0.3\libraries\DateTime\DateTime.cpp: In member function 'void DateTimeClass::setTime(time_t)':
C:\Users\Will\Downloads\Arduino\arduino-1.0.3\libraries\DateTime\DateTime.cpp:28: error: 'millis' was not declared in this scope
C:\Users\Will\Downloads\Arduino\arduino-1.0.3\libraries\DateTime\DateTime.cpp: In member function 'time_t DateTimeClass::now()':
C:\Users\Will\Downloads\Arduino\arduino-1.0.3\libraries\DateTime\DateTime.cpp:43: error: 'millis' was not declared in this scope
Any ideas?
Thanks,
Will
Having exact same issue ... :-(
Delete(aldo i'm using arduino-1.5.2)
any idea's/solutions?
solved the issue.. from arduino web site you can download time.h lib .. that one works!
DeleteI only added #include <Arduino.h. within the date.time file as mentioned above. It works fine.
DeleteThis comment has been removed by the author.
ReplyDeleteThis comment has been removed by the author.
DeleteCiao, sketch funzionante alla perfezione, peccato non si aggiorni l'anno, resta sempre 2010. Avete qualche suggerimento in merito?
ReplyDeleteCordiali saluti
Cris
problem: Datetime is not declared in this scope..
ReplyDeletewhat should i do???
So any ideas on this? Here's the error I get.
ReplyDeleteC:\Arduino\libraries\DateTime\DateTime.cpp: In member function 'void DateTimeClass::setTime(time_t)':
C:\Arduino\libraries\DateTime\DateTime.cpp:28: error: 'millis' was not declared in this scope
C:\Arduino\libraries\DateTime\DateTime.cpp: In member function 'time_t DateTimeClass::now()':
C:\Arduino\libraries\DateTime\DateTime.cpp:43: error: 'millis' was not declared in this scope
Any ideas? Thank you.
me too:
DeleteArduino: 1.5.5-r2 (Windows 8), Board: "Arduino Uno"
C:\Users\Steve\Documents\Arduino\libraries\DateTime\DateTime.cpp: In member function 'void DateTimeClass::setTime(time_t)':
C:\Users\Steve\Documents\Arduino\libraries\DateTime\DateTime.cpp:28: error: 'millis' was not declared in this scope
C:\Users\Steve\Documents\Arduino\libraries\DateTime\DateTime.cpp: In member function 'time_t DateTimeClass::now()':
C:\Users\Steve\Documents\Arduino\libraries\DateTime\DateTime.cpp:43: error: 'millis' was not declared in this scope
This report would have more information with
"Show verbose output during compilation"
enabled in File > Preferences.
Follow this link for solution
ReplyDeletehttp://www.frustration.in/blog/archives/181
Thanks for the info, rally helps in repairing DateTime.cpp.
Deletemaybe that is why its already superseded by Time.h
Anyway, the Year seem stuck with 2010
What would be the solution for following error ??
ReplyDeleteArduino: 1.6.5 (Windows 8.1), Board: "Arduino/Genuino Uno"
In file included from Clock.ino:11:0:
D:\arduino-1.6.5-r5\hardware\arduino\avr\cores\arduino/Arduino.h:117:14: error: conflicting declaration 'typedef bool boolean'
typedef bool boolean;
^
In file included from Clock.ino:2:0:
D:\arduino-1.6.5-r5\libraries\DateTime/DateTime.h:17:17: error: 'boolean' has a previous declaration as 'typedef uint8_t boolean'
typedef uint8_t boolean;
^
Error compiling.
This report would have more information with
"Show verbose output during compilation"
enabled in File > Preferences.
What would be the solution for following error ??
ReplyDeleteArduino: 1.6.5 (Windows 8.1), Board: "Arduino/Genuino Uno"
In file included from Clock.ino:11:0:
D:\arduino-1.6.5-r5\hardware\arduino\avr\cores\arduino/Arduino.h:117:14: error: conflicting declaration 'typedef bool boolean'
typedef bool boolean;
^
In file included from Clock.ino:2:0:
D:\arduino-1.6.5-r5\libraries\DateTime/DateTime.h:17:17: error: 'boolean' has a previous declaration as 'typedef uint8_t boolean'
typedef uint8_t boolean;
^
Error compiling.
This report would have more information with
"Show verbose output during compilation"
enabled in File > Preferences.